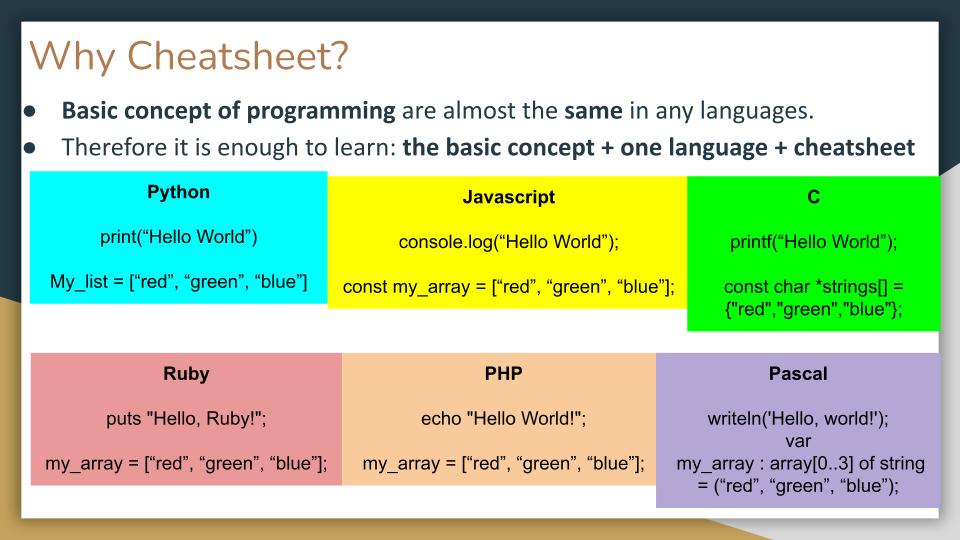
Table Of Contents
Frequently Used In Any Programming
Print
Comments
Variables
Data Types
x = 1 #integer
y = 2.5 #float
z = "My numbers are:" #string
print(z, x, "and", y)
Operators
x = 5
y = 2.5
z = x + y #addition
print("x + y =", z)
z = x - y #substraction
print("x - y =", z)
z = x * y #multiplication
print("x * y =", z)
z = x / y #division
print("x / y =", z)
z = x % y #mod
print("x % y =", z)
Input
print('Enter your name:')
x = input()
print('Hello, ' + x)
Boolean
x = 1;
y = 2;
z = "hello"
# more than?
v = x > y
print(x, ">", y, ":", v)
# less than?
v = x < y
print(x, "<", y, ":", v)
# equal?
print("hello =", z, ":", ("hello" == z))
Conditional
grade = 75
if grade > 60:
print("pass")
if grade < 60:
print("fail")
else:
print("pass")
if grade <= 0:
print("F")
elif grade <= 20:
print("E")
elif grade <= 40:
print("D")
elif grade <= 60:
print("C")
elif grade <= 80:
print("B")
elif grade <= 100:
print("A")
else:
print("Not a Number")
Loop
for x in [0, 1, 2, 5, 8]:
print("interation:", x)
for x in range(0, 4):
print(x)
for x in range(0, 4):
print("hello")
y = 1
z = 0
# Find y + y + y + y!
for x in range(0, 4):
z += y
print(z)
List (Array)
colors = ["black", "white", "red", "green", "blue"]
print(colors[0])
print(colors[2])
print(colors[4])
print(len(colors))
for x in range(0, len(colors)):
print(colors[x])
Dictionaries (objects)
profile = {
"Name": "Fajar Purnama",
"Age": 30,
"Nationality": "Indonesian",
"Occupation": "Lecturer"
}
print(profile)
print(profile["Name"])
print(profile["Nationality"])
for x in profile:
print(x, ":", profile[x])
Navigate Independently
Show available built in functions
Show description of a function
Show available modules
List functions available in a module
Install modules
!pip install pydataset
Exercises
References